번호판 인식 프로젝트
(참고: https://velog.io/@mactto3487/프로젝트-OpenCV-자동차-번호판-인식)
OpenCV 자동차 번호판 인식
자동차 번호판 인식 with OpenCV 1. 라이브러리 호출 2. Read Input Image 3. Convert Image to Grayscale¶ 4. Adaptive Thresholding 4-1. Gaussian Blur 비적용 / 적용 비교 5.
velog.io
프로젝트 계획
1. 번호판 인식 프로그램
- OpenCV를 활용하여 번호판 인식 프로그램 설계
- 카메라가 필요할 수도 있음, 없다면 이미지 파일로 대체
- 최대한 높은 인식률 목표
- 인식 후에 데이터를 pandas를 활용하여 csv파일로 저장
2. 번호판 등록 - 데이터 베이스 설계
- csv파일에 있는 번호판 데이터 전처리 후 db에 저장
- 가상의 번호판 데이터 베이스 생성
- 스키마 설계
- SQlite or MySQL 사용
3. 관리 프로그램 - GUI 구현
- 이미지를 불러와서 번호판 인식
- 인식한 번호판을 DB에서 조회해서 DB에 있다면 Pass, 없으면 Fail
4. 아두이노/서버 모터 사용 차단기 개발?!
- 이건 그냥 간단하게 초음파 센서 사용해서 거리가 가까워지면 서브 모터 사용해서 차단기 올릴 예정
1-1. 번호판 인식 프로그램
import cv2
import pytesseract
pytesseract.pytesseract.tesseract_cmd = 'C:/Program Files/Tesseract-OCR/tesseract'
# 이미지 파일 읽기
image = cv2.imread('img/님 이미지 파일.jpg')
cv2.imshow("Original",image)
# 회색 이미지로 전환
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
#캐니 엣지 탐지 사용
canny_edge = cv2.Canny(gray_image, 170, 200)
# 엣지를 기준으로 윤곽 찾기
contours, new = cv2.findContours(canny_edge.copy(), cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)
contours=sorted(contours, key = cv2.contourArea, reverse = True)[:30]
# 번호판 등고선 및 x,y,w,h 좌표 초기화
contour_with_license_plate = None
license_plate = None
x = None
y = None
w = None
h = None
# 4개의 잠재적인 모서리가 있는 윤곽선을 찾고 그 주변에 ROI 생성
for contour in contours:
# 등고선 둘레 찾기, 닫힌 등고선이어야 한다
perimeter = cv2.arcLength(contour, True)
approx = cv2.approxPolyDP(contour, 0.01 * perimeter, True)
if len(approx) == 4:
contour_with_license_plate = approx
x, y, w, h = cv2.boundingRect(contour)
license_plate = gray_image[y:y + h, x:x + w]
break
(thresh, license_plate) = cv2.threshold(license_plate, 127, 255, cv2.THRESH_BINARY)
cv2.imshow("plate",license_plate)
# Tesseract에 이미지를 보내기 전에 노이즈 제거
license_plate = cv2.bilateralFilter(license_plate, 11, 17, 17)
(thresh, license_plate) = cv2.threshold(license_plate, 150, 180, cv2.THRESH_BINARY)
# 텍스트 인식 (한글을 인식할 수 있게 언어를 한국어로 설정)
text = pytesseract.image_to_string(license_plate, lang='kor')
# 차량번호판을 그리고 텍스트 쓰기
image = cv2.rectangle(image, (x,y), (x+w,y+h), (0,0,255), 3)
image = cv2.putText(image, text, (x-100,y-20), cv2.FONT_HERSHEY_SIMPLEX, 1, (0,255,0), 2, cv2.LINE_AA)
print("License Plate :", text)
cv2.imshow("License Plate Detection",image)
cv2.waitKey(0)
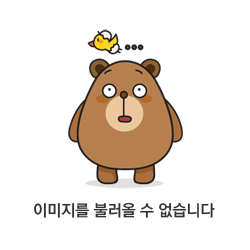
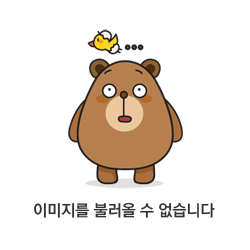
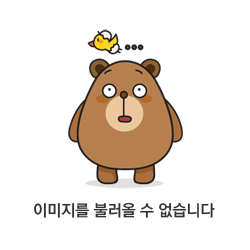
License Plate : 、 123 허 3456
이렇게 잘 인식하기는 하는데 " 、 123 허 3456 " 이런 식으로 다른 텍스트가 들어가 있는 것을 볼 수 있다. 이런 건 추후에 db에 넣기 전에 데이터 전처리를 통해서 수정하면 되니 지금은 스킵한다.
번호판을 인식되는 걸 확인했으니 이제 img폴더에 있는 모든 이미지를 인식해서 csv파일로 저장해 보자
import os
import cv2
import pytesseract
import pandas as pd
# 경로 설정
pytesseract.pytesseract.tesseract_cmd = 'C:/Program Files/Tesseract-OCR/tesseract'
image_folder_path = 'img/' # 이미지가 있는 폴더 경로
output_csv_file = 'car_num.csv' # 결과를 저장할 CSV 파일 경로
# 번호판 텍스트 추출 함수
def extract_license_plate_text(image_path):
image = cv2.imread(image_path)
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
canny_edge = cv2.Canny(gray_image, 170, 200)
contours, _ = cv2.findContours(canny_edge.copy(), cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)
contours = sorted(contours, key=cv2.contourArea, reverse=True)[:30]
for contour in contours:
perimeter = cv2.arcLength(contour, True)
approx = cv2.approxPolyDP(contour, 0.01 * perimeter, True)
if len(approx) == 4:
x, y, w, h = cv2.boundingRect(contour)
license_plate = gray_image[y:y + h, x:x + w]
(thresh, license_plate) = cv2.threshold(license_plate, 127, 255, cv2.THRESH_BINARY)
license_plate = cv2.bilateralFilter(license_plate, 11, 17, 17)
(thresh, license_plate) = cv2.threshold(license_plate, 150, 180, cv2.THRESH_BINARY)
text = pytesseract.image_to_string(license_plate, lang='kor')
return text.strip()
return None
# 모든 이미지 파일에서 번호판 텍스트 추출
results = []
for filename in os.listdir(image_folder_path):
if filename.endswith(('.png', '.jpg', '.jpeg')):
image_path = os.path.join(image_folder_path, filename)
text = extract_license_plate_text(image_path)
results.append({'Image': filename, 'License Plate': text})
# 결과를 CSV 파일로 저장
df = pd.DataFrame(results)
df.to_csv(output_csv_file, index=False)
print("CSV 파일이 성공적으로 생성되었습니다:", output_csv_file)
정상적으로 코드를 실행시키면 car_num.csv 파일이 생성된 걸 볼 수 있다.
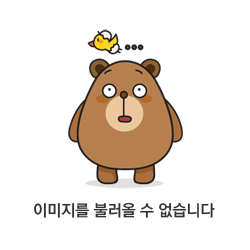
Source Code: https://github.com/jackpraveenraj/Number-Plate-Recognition-Using-OpenCV
GitHub - jackpraveenraj/Number-Plate-Recognition-Using-OpenCV: A fully functioning Number Plate Recognition Software made using
A fully functioning Number Plate Recognition Software made using Python (Pytesseract and Machine Learning). The software allows users to detect moving vehicles, separate the number plate and detect...
github.com
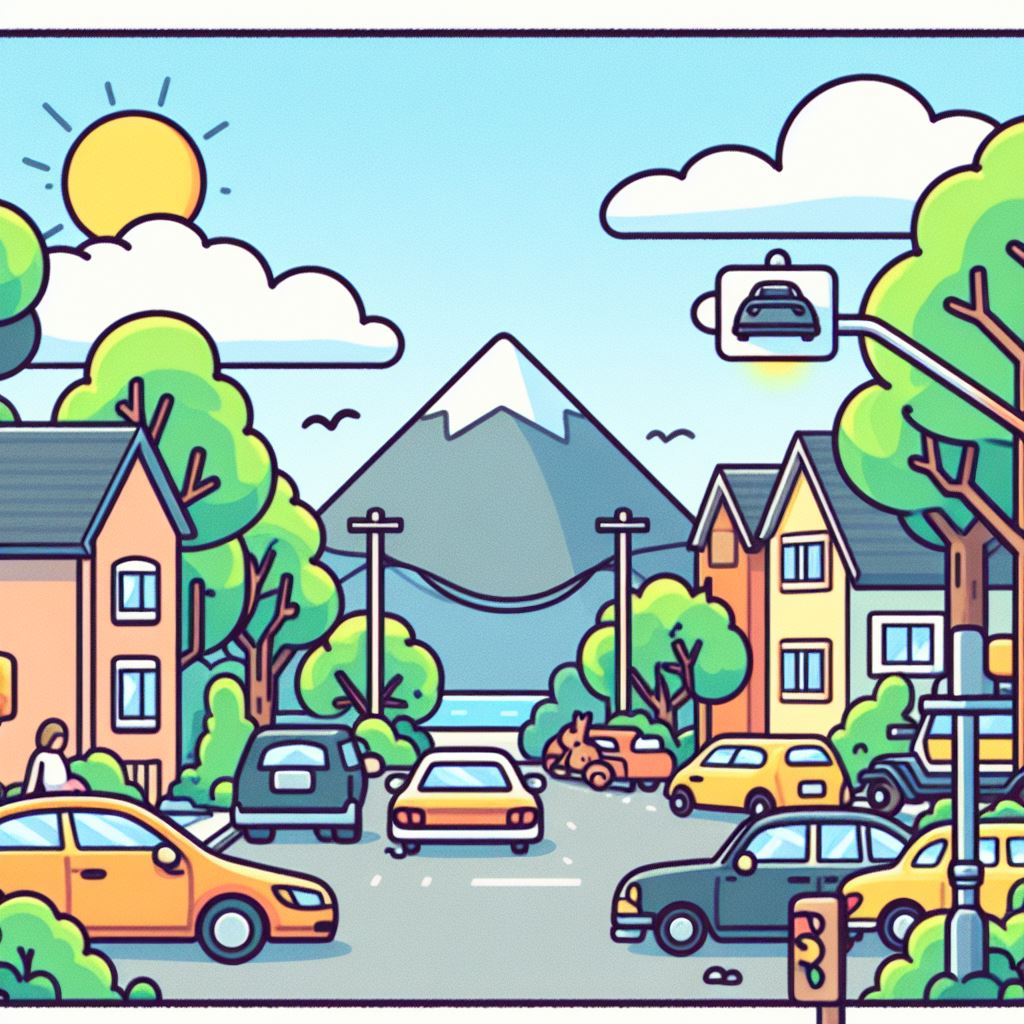
'AIoT' 카테고리의 다른 글
AIoT 정규 64일차 (0) | 2024.04.11 |
---|---|
AIoT 정규 63일차 (0) | 2024.04.09 |
AIoT 정규 61일차 (0) | 2024.04.05 |
AIoT 정규 60일차 - Git (0) | 2024.04.04 |
AIoT 정규 59일차 (1) | 2024.04.03 |